アプリケーション各処理の説明
クラスの全体的な構成の説明が終わったところで、アプリケーションの仕様に従って、商品データ(商品XML)のデータ処理について説明していきます。
商品データ(商品XML)新規作成処理
まずはXMLの新規作成処理について説明します。
画面遷移
商品情報 新規登録の画面遷移を以下の図に示します。
「商品情報 新規登録」画面は、商品一覧の新規登録ボタンを押下すると表示されます。「商品情報 新規登録」画面で商品情報を入力し「新規登録」ボタンを押下すると、XMLDBに商品データが新規XMLとして作成されます。
「商品情報 新規登録」画面の表示処理は、ただ単にJSPを表示するだけですので、説明は割愛し「新規登録」ボタンが押下された場合のXMLデータ保存処理について説明します。
コントローラ(ItemCRUDServlet)
コントローラとなるサーブレットでは、HTMLから「action="create"」というパラメータを受け取り、以下の順で、XMLデータ新規作成処理を実行します。
- 画面入力されたリクエストパラメータを元にItemクラスを生成し値を格納する(
createItem
メソッド) - ItemDaoの
create
メソッドを呼び出す - 商品一覧画面(list.jsp)にForwardする
以下にItemCRUDServletのソースを示します。
public class ItemCRUDServlet extends HttpServlet { /** サーブレットから呼び出すDao */ private ItemDao itemDao = null; /** サーブレットの初期化処理 */ public void init(ServletConfig config) throws ServletException{ super.init(); itemDao = ItemDaoFactory.createDao(); } /** doGet、doPostの両メソッドから呼ばれる共通メソッド */ private void doService(HttpServletRequest req, HttpServletResponse res){ String action = req.getParameter( "action" ); // ~省略 }else if (action.equals("create")) { // 新規登録処理 Item item = createItem( req ); try { itemDao.create( item ); } catch (Exception e) { e.printStackTrace(); forwardToErrorPage(req, res); return; } forwardToList(req, res); } /** リクエストパラメータを元にItemのインスタンスを生成 */ private Item createItem(HttpServletRequest req){ Item item = new Item(); item.setItemCode( req.getParameter("item_code") ); item.setItemName( req.getParameter("item_name") ); item.setPrice( Integer.parseInt( req.getParameter( "price" ) ) ); Description desc = new Description(); desc.setCaption( req.getParameter("caption") ); desc.setHeader( req.getParameter("header") ); desc.setFooter( req.getParameter("footer") ); item.setDescription( desc ); item.setOptionName( req.getParameter("option_name") ); String option1 = req.getParameter("option1"); item.addOption( (option1==null) ? "" : option1 ); String option2 = req.getParameter("option2"); item.addOption( (option2==null) ? "" : option2 ); String option3 = req.getParameter("option3"); item.addOption( (option3==null) ? "" : option3 ); item.setRegDate(new Date() ); return item; }
init
メソッド、doServiceの記述は省略します。Daoクラス
ItemDaoImplクラス
ItemDaoImplクラスのcreate
メソッドでは、以下の2つの処理を行っています。
- LuxeonDaoクラスのcreateXMLを呼び出しXMLを新規作成
- LuxeonDaoクラスのaddDocumentToBinderを呼び出し新規に作成したXMLをバインダドキュメントに追加
以下にItemDaoImplクラスのcreate
メソッドのソースを示します。
public void create(Item item) throws JAXBException,IOException{ String xmlName = "/items/" + item.getItemCode() + ".xml"; LuxeonDao.createXML( item , xmlName ); LuxeonDao.addDocumentToBinder( "items/item_container.bnd" , xmlName ); }
LuxeonDaoクラスのcreateXML
メソッドの第1引数には、サーブレットで画面入力値を元に生成したItemクラスのインスタンス、第2引数には、Cyber Luxeon上のXMLのファイル名を渡しています。XMLを保存するパス名のルールは「XMLStoreのルートディレクトリからのパス(/items)/商品コード(Cen001).xml」です。また、XML作成後、バインダドキュメントにXMLへのリンクを設定しています。こうすることにより、複数のXMLを束ねて1つのXMLとして検索する処理を実現しています。
以下の図は、新規登録処理後に作成したXMLをDXE Managerで参照した画面です。
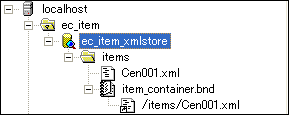
新規に作成したXML(items/Cen001.xml)とバインダドキュメント(item_container.bnd)とバインダドキュメント上に作成されたリンクが確認できます。
LuxeonDaoクラス
次にItemDaoImplクラスのcreate
メソッドから呼ばれるLuxeonDaoクラスのcreateXML
メソッドの説明をします。LuxeonDaoクラスはCyber LuxeonのJava APIとJAXB2.0を使用して実際にXMLをDBに保存する処理を以下の順で実行しています。
- セッション、XMLStore、ルートディレクトリを順に取得
- XMLをDB上に新規作成し、XMLへの出力ストリームを取得
- JAXBでItemクラスのインスタンスをXMLに変換しXMLの出力ストリームに出力
- XMLの出力ストリーム、セッションを閉じる
以下にLuxeonDaoクラスのcreateXML
メソッドのソースを示します。
/** クラス変数:XMLストア名 */ private static final String XML_STORE_NAME = "ec_item_xmlstore"; /** クラス変数:出力XMLの文字エンコーディング*/ private static final String XML_ENCODING = "UTF-8"; public static void createXML( Object obj ,String xmlName ) throws IOException, JAXBException , XlnSessionException{ Session clientSession = null; try { // セッションの取得 clientSession = XlnClientSessionFactory.getSession(); XMLStore xmlStore = clientSession.getXMLStore( XML_STORE_NAME ); Directory dir = xmlStore.getRootDirectory(); // XMLドキュメントの生成 XMLDocument xmlDoc = dir.createXMLDocument( xmlName ); XlnOutputStream xlnOutputStream = null; try { xlnOutputStream = xmlDoc.getOutputStream(); xlnOutputStream.setContentType( "text/xml" ); xlnOutputStream.setOverwrite( XlnOutputStream.OVERWRITE ); // JAXBを使用して、オブジェクト→XML変換 JAXBContext context = JAXBContext.newInstance( obj.getClass() ); Marshaller marshaller = context.createMarshaller(); marshaller.setProperty( Marshaller.JAXB_ENCODING, XML_ENCODING ); marshaller.setProperty( Marshaller.JAXB_FORMATTED_OUTPUT, Boolean.FALSE ); // 変換 marshaller.marshal( obj , xlnOutputStream ); }finally{ if ( xlnOutputStream != null ){ xlnOutputStream.close(); } } } finally{ if ( clientSession != null ) clientSession.release(); } }