コードからEFを利用する
準備が完了したので、SalesforceContextを使ってデータを取得するModelクラスを作成します。
Imports System.Collections.ObjectModel Imports System.ComponentModel Imports System.Runtime.CompilerServices Namespace Models Public Class SalesForceModel Implements INotifyPropertyChanged Public Property Items As New ObservableCollection(Of Campaign) Public Sub GetCampaign() Dim ents = New SalesforceContext() Dim campaignQuery = From item In ents.Campaign Order By item.Id Select item Me.Items = New ObservableCollection(Of Campaign)(campaignQuery) OnPropertyChanged("Items") End Sub Public Event PropertyChanged(sender As Object, e As PropertyChangedEventArgs) _ Implements INotifyPropertyChanged.PropertyChanged Private Sub OnPropertyChanged(<CallerMemberName> _ Optional propertyName As String = Nothing) RaiseEvent PropertyChanged(Me, New PropertyChangedEventArgs(propertyName)) End Sub End Class End Namespace
SalesforceContextはLINQで操作できる形のオブジェクトになるので、取得後にLINQを使って並び替えを行っています。その後、ObservableCollection型のItemsプロパティに設定し画面からBindingにより参照できるようにしておきます。
Binding用ViewModel
ModelとViewを連携するためのViewModelを作成します。
Imports System.ComponentModel Imports System.Runtime.CompilerServices Imports CZ1411Spread.Models Imports System.Collections.ObjectModel Imports CZ1411Spread.Common Namespace ViewModels Public Class MainViewModel Implements INotifyPropertyChanged Private WithEvents Model As New SalesForceModel Public Property Items As ObservableCollection(Of Campaign) Get Return Me.Model.Items End Get Set(value As ObservableCollection(Of Campaign)) Me.Model.Items = value End Set End Property Private _GetCommand As RelayCommand Public Property GetCommand() As RelayCommand Get If _GetCommand Is Nothing Then _GetCommand = New RelayCommand(AddressOf Me.GetCampaign) End If Return _GetCommand End Get Set(value As RelayCommand) _GetCommand = value End Set End Property Public Sub GetCampaign() Dim userID = "hatsune@wankuma.com" Dim password = "hogehoge" Me.Model.GetCampaign(userID, password) End Sub Private Sub Model_PropertyChanged(sender As Object, … OnPropertyChanged(e.PropertyName) End Sub Public Event PropertyChanged(sender As Object, … Private Sub OnPropertyChanged(<CallerMemberName> Optional propertyName … RaiseEvent PropertyChanged(Me, New PropertyChangedEventArgs(propertyName)) End Sub End Class End Namespace
GridViewを使ったView
ObservableCollectionをGridViewに表示するViewを定義します。
<Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:sg="http://schemas.grapecity.com/windows/spreadgrid/2012" x:Class="Views.MainWindow" Title="CZ1412Salesforce" Height="300" Width="498"> <Grid> <Grid.RowDefinitions> <RowDefinition Height="*"/> <RowDefinition Height="Auto"/> </Grid.RowDefinitions> <DataGrid HorizontalAlignment="Left" VerticalAlignment="Top" ItemsSource="{Binding Items}" CanUserSortColumns="True" /> <StackPanel Grid.Row="1" Orientation="Horizontal" Margin="10"> <Button Content="GET" Command="{Binding GetCommand}" /> </StackPanel> </Grid> </Window>
実行結果
このサンプルを実行すると、データが表示されていない画面が表示されます。[GET]ボタンをクリックすると、ViewModelのGetCommandが実行されてデータの取得が行われます。
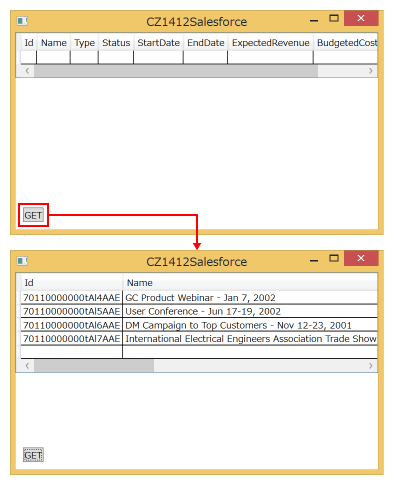
まとめ
EntityFramworkを利用することでADO.NETインターフェースのConnectionやCommand、データセットなどを意識する必要がなくなります。共通クラスとしてデータアクセス部分を隠蔽することで意図しないSQLの発行を未然に防ぐことができますし障害時の切り分けや対応もしやすくなります。ある程度以上の規模になるとEntityFrameworkは必要な手法になるので、CDataとあわせて効率よい開発を実現してみてはいかがでしょうか。