Torinoのインストール
Visual C++ Compiler november 2013 CTP:Torinoはhttp://aka.ms/Icp591からダウンロードできます。Visual Studio 2013(Express版でもOK)のインストールされたマシン上にTorinoをインストールすると、Visual C++プロジェクトのプロパティ/プラットフォームツールセットに"Visual C++ Compiler Nov 2013 CTP(CTP_Nov2013)"が追加されます。
TorinoはVisual Studio IDE本体には手を触れず、C++コンパイラ、ヘッダ、ライブラリのみを追加します。
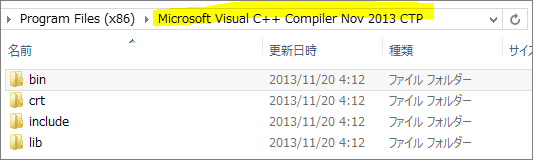
インテリセンスは変更されないので、これから紹介する新しい構文に対応できずあちこちに赤い波線が現れること、なにとぞご承知おきください。
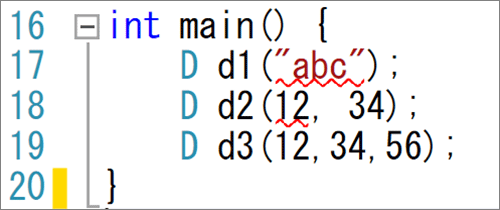
前準備はここまで。Torinoで追加された主要新機能、一気にいきますよ。
extended sizeof
昔からあるコンパイル時演算子:sizeofが拡張され、struct/classのメンバに対してsizeofできるようになりました。
#include <iostream> using namespace std; struct X { char c; protected: int i; private: long l; }; int main() { cout << sizeof(X::c) << endl; cout << sizeof(X::i) << endl; // protected/private メンバの cout << sizeof(X::l) << endl; // 大きさもわかるね! }
今までできなかったのが不思議なくらいです。
non-static data member initialization
これもまた、なんで今までできなかったの?な機能です。staticでないデータメンバの初期化は、従来ならコンストラクタで行っていましたが、初期値を宣言部に書きおろすことができます。
#include <iostream> #include <string> struct X { int a = 123; std::string b = "four"; }; using namespace std; int main() { X x; cout << x.a << ':' << x.b << endl; }
ただし、まだちょっと不具合があるみたい。std::vector<int> c { 1, 2, 3 }; はエラーになっちゃいました。単純な初期化ならほぼ大丈夫なようです。
inheriting constructor
とあるクラスから新たなクラスを導出するとき、コンストラクタの定義が面倒なことがままあります。基底クラスのコンストラクタをそのまま使えばいい場合、コンストラクタの"横流し"をせっせと用意せにゃなりません。
#include <iostream> using namespace std; struct B { B() = delete; explicit B(const char*) { cout << "B::B(const char*)\n"; } B(int, int) { cout << "B::B(int,int)\n"; } }; struct D : B { D(const char* arg) : B(arg) {} // 基底クラスのコンストラクタに横流し D(int arg0, int arg1) : B(arg0, arg1) {} // 同上 D(int, int, int) : B("3-args") { cout << "D::D(int,int,int)\n"; } };
Torinoなら、基底クラスのコンストラクタをそのまま継承することができます。
struct B { B() = delete; explicit B(const char*) { cout << "B::B(const char*)\n"; } B(int, int) { cout << "B::B(int,int)\n"; } }; struct D : B { using B::B; // B(const char*), B(int,int) を継承する D(int, int, int) : B("3-args") { cout << "D::D(int,int,int)\n"; } };