ビットマップの使用方法
ビットマップは、画面各部のコピーやスクロールに使います。CBitmap
クラスはビットマップオブジェクトをカプセル化したもので、ビットマップを操作するための機能を備えています。
ビットマップの使用例1
次に示す1番目の例では、ウィンドウのデバイスコンテキストにビットマップを描画します。このためには、次の手順を実行します。
- リソースからビットマップを読み込みます。
- BITMAPオブジェクトを使ってビットマップに関する情報(幅、高さなど)を取得します。
- ペイントデバイスコンテキストと互換性のあるメモリデバイスコンテキストを作成します(CreateCompatibleDCを参照)。
- ビットマップをこのメモリデバイスコンテキストに切り替えます。
- メモリデバイスコンテキストのビットマップをペイントデバイスコンテキストにコピーします(BitBltを参照)。
void CChildView::OnPaint() { CPaintDC dc(this); CRect rc; GetClientRect(rc); // load the bitmap from the resources CBitmap bmp; bmp.LoadBitmap(IDB_MARIUS); // get information about the bitmap BITMAP tagbmp; bmp.GetBitmap(&tagbmp); // create a in-memory device context, // compatible with the painting device context CDC memDC; memDC.CreateCompatibleDC(&dc); // select the loaded bitmap into the in-memory device context CBitmap* oldbitmap = (CBitmap*)memDC.SelectObject(&bmp); //copy the bitmap from the in-memory DC to the painting DC dc.BitBlt( // position on the destination: center (rc.Width() - tagbmp.bmWidth)/2, (rc.Height() - tagbmp.bmHeight)/2, // height of the bitmap to copy: same as loaded bitmap tagbmp.bmWidth, tagbmp.bmHeight, // source device context: in-memory DC &memDC, // position from the source: top-left corner 0, 0, // raster operation to perform: direct copy SRCCOPY); // select the old rectangle back memDC.SelectObject(oldbitmap); }
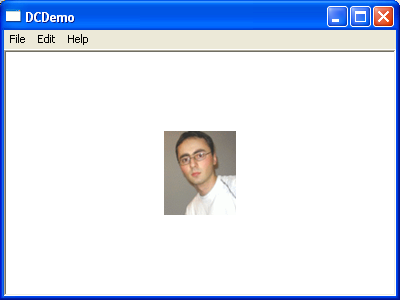
ビットマップの使用例2
CreateBitmap()
を使ってビットマップをプログラムで作成することもできます。次の例では、幅1ピクセル、高さ255ピクセル、32ビットのビットマップを作成します。各ピクセルの色はRGB(255, 255, 255)から開始し、RGB(0, 0, 0)で終了します。これを使って、四角形を塗りつぶすグラデーションのパターンブラシを作成します。
void CChildView::OnPaint() { CPaintDC dc(this); CRect rc; GetClientRect(rc); rc.DeflateRect(50, 50, 50, 50); // creat an array of 255 colors int segments = 255; DWORD* dwBits = new DWORD[segments]; for(int i = 0; i < segments; ++i) { dwBits[i] = (DWORD)RGB(0xFF - i, 0xFF - i, 0xFF - i); }; // create a 32-bit bitmap of 1 pixel width and 255 pixels // height HBITMAP hBitmap = ::CreateBitmap(1, segments, 1, 32, dwBits); delete [] dwBits; // create a pattern brush CBrush brush; brush.CreatePatternBrush(CBitmap::FromHandle(hBitmap)); CBrush* oldbrush = (CBrush*)dc.SelectObject(&brush); // draw a rectangle with the gradient pattern brush dc.Rectangle(rc); dc.SelectObject(oldbrush); }
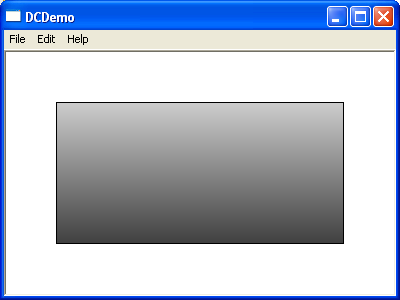
フォントの使用方法
CFont
クラスはフォントをカプセル化したもので、フォントを操作するときに使います。フォントはテキストの描画に使われます。CDC
クラスには、テキストを描画するための2つのメソッドがあります。
- DrawText
テキストを書式設定し、指定の四角形内に描画します - DrawTextEx
追加の書式設定オプションを提供します
次の例では、高さ16ピクセルのArialフォントを作成します(CreateFontに対して指定する値の単位は論理単位です。そのため、ピクセルから論理単位への変換を行う必要があります)。このフォントを使って、ウィンドウのクライアント領域の中央(垂直方向と水平方向)に1行のテキストを描画します。
void CChildView::OnPaint() { CPaintDC dc(this); CRect rc; GetClientRect(rc); // compute the hight in logical units int height = -MulDiv(16, dc.GetDeviceCaps(LOGPIXELSY), 72); // create the font CFont font; font.CreateFont( height, // nHeight 0, // nWidth 0, // nEscapement 0, // nOrientation FW_NORMAL, // nWeight FALSE, // bItalic FALSE, // bUnderline 0, // cStrikeOut ANSI_CHARSET, // nCharSet OUT_DEFAULT_PRECIS, // nOutPrecision CLIP_DEFAULT_PRECIS, // nClipPrecision DEFAULT_QUALITY, // nQuality DEFAULT_PITCH | FF_SWISS, // nPitchAndFamily _T("Arial")); // select the font into the device context CFont* oldfont = (CFont*)dc.SelectObject(&font); // set the color to red COLORREF oldcolor = dc.SetTextColor(RGB(255, 0, 0)); // draw the text dc.DrawText( // text _T("This is a sample text"), // rectangle in which to draw the text rc, // center the text in the middle of the rectangle DT_CENTER|DT_VCENTER|DT_SINGLELINE); // select the old font dc.SelectObject(oldfont); }
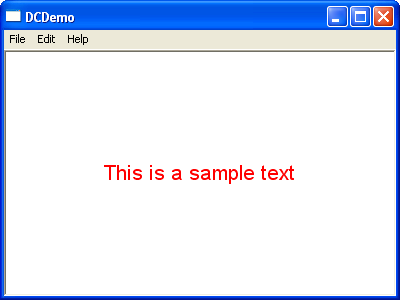
まとめ
本稿では線の描画、ブラシによるシェイプの塗りつぶし、ビットマップの表示、テキストの描画など、MFCでの基本的なグラフィック操作について説明しました。本稿が入門編として参考になれば幸いです。